The translation of the article was prepared specifically for students of the course "Developer on the Spring Framework" .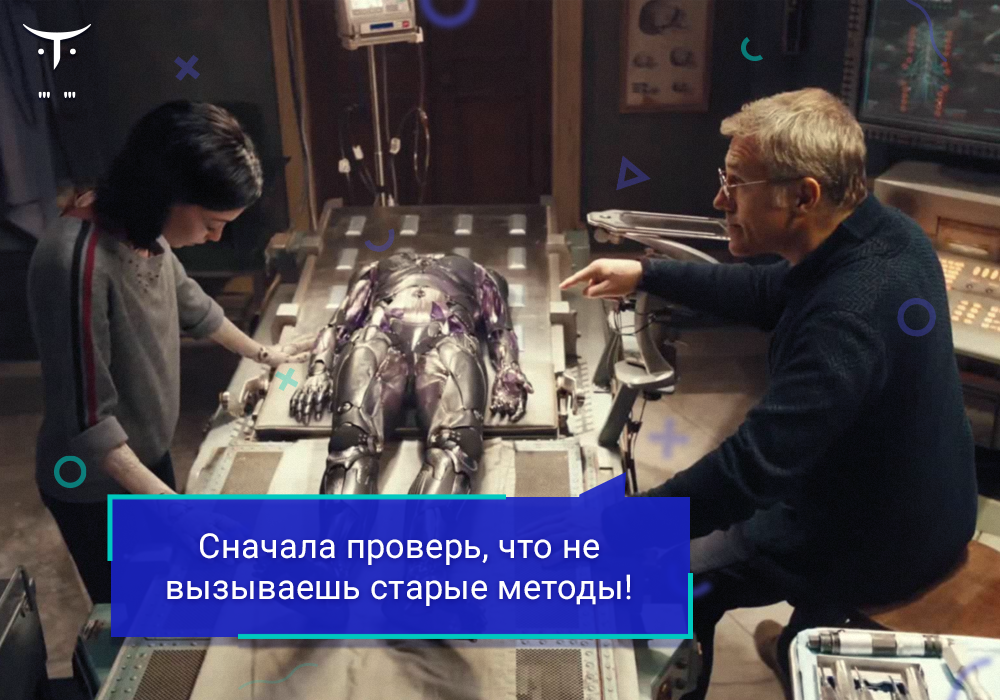
On October 16, 2019, Spring Boot 2.2 was released!
In this post you will learn about the many new goodies that version 2.2 offers you.
Maven
To get started with Spring Boot 2.2. In your application, upgrade to the new version of
starter-parent .
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.2.0.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>com.example</groupId> <artifactId>your-very-cool-project</artifactId> <version>0.0.1-SNAPSHOT</version> <name>your-very-cool-project</name> <description>Spring Boot 2.2 Project</description> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> </dependencies> </project>
If you are starting the application from scratch, create a Maven or Grade project at
start.spring.io !

Global lazy bean initialization
Lazy initialization of bean components has long been supported in the Spring Framework.
The lazily initialized bean tells the IoC container to instantiate the bean at the first request, and not at startup.
The new feature introduced in Spring Boot 2.2 is support for the global
lazy initialization of bean components (this feature is disabled by default).
What happens when you turn on global lazy bean initialization?
- Initialization of all Spring beans and their dependencies will be delayed until they are needed.
To reduce application startup time, you can now enable the global lazy initialization of Spring beans in the configuration properties using:
spring.main.lazy.initialization=true
or for yml configuration:
spring: main: lazy-initialization: true
Are there any disadvantages to using lazy bean initialization? Of course! It is very important to understand the consequences - you should not include the global initialization of bean components without thinking about this solution! There are some tradeoffs to consider:
- Processing HTTP requests may take longer while any lazy initialization occurs. Subsequent requests are not affected.
- Failures that usually occur at startup (since Spring beans and their dependencies are created when creating the application context) will now not occur immediately. Thus, your application will no longer produce obvious startup crashes! As a result: your customer may be the first to encounter a problem (bean wiring).
If you do not want to enable lazy initialization of the bean (
spring.main.lazy.initialization = false
) globally, you can consider setting up lazy initialization for a particular component using the
@Lazy
annotation.
On the other hand, it is also possible to enable lazy initialization of bean components (
spring.main.lazy.initialization = true
) at the global level and explicitly disable lazy initialization for a particular component.
Summarize:

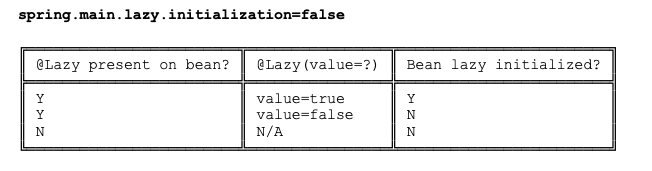
JMX is disabled by default
Starting with Spring Boot 2.2, JMX is disabled by default. This helps reduce application startup time and not waste a significant amount of resources at runtime. If you are still dependent on JMX, you can enable it again:
spring.jmx.enabled=true
or:
spring: jmx: enabled: true
Improvements for configuration properties
Spring Boot 2.2 comes with some nice improvements for configuration properties.
@ConfigurationProperties
scan support for @ConfigurationProperties
- Immutable binding
@ConfigurationProperties
Classpath scan support for @ConfigurationProperties
Spring Boot will create a bean for each configuration class annotated with
@ConfigurationProperties
found when scanning the
classpath . This is an alternative to using
- @EnableConfigurationProperties for a class that binds a property class
- or using the
@Component
annotation in the configuration class to make it a bean.
Keep in mind that two bean components can be created for the configuration class, annotated with both
@Component
and
@ConfigurationProperties
. In such cases,
@Component
should be removed from your configuration class!
Immutable binding @ConfigurationProperties
Using constructor-based properties binding now supports immutable configuration property classes. Constructor-based
@ConfigurationProperties
can be enabled by annotating the
@ConfigurationProperties
class or one of its constructors with
@ConstructorBinding
.
For example:
package com.example.immutable.configuration.binding; import lombok.Getter; import org.springframework.boot.context.properties.ConfigurationProperties; import org.springframework.boot.context.properties.ConstructorBinding; @Getter @ConfigurationProperties("stock.quote.subscription") public class ImmutableStockQuoteSubscriptionProperties { private final String endpoint; private final String apiKey; private final SubscriptionType subscriptionType; private final boolean enabled; @ConstructorBinding public ImmutableStockQuoteSubscriptionProperties(String endpoint, String apiKey, SubscriptionType subscriptionType, boolean enabled) { this.endpoint = endpoint; this.apiKey = apiKey; this.subscriptionType = subscriptionType; this.enabled = enabled; } enum SubscriptionType { REALTIME, DELAYED, } }
properties in my application.yml:
stock: quote: subscription: endpoint: http:
See the
documentation for more details.
Actuator Endpoint Changes
The
/actuator/health
endpoint changed the resulting JSON format by renaming
details
to
components
for first-level elements.
The actuator media type has been changed from:
application/vnd.spring-boot.actuator.v2+json
to
application/vnd.spring-boot.actuator.v3+json
.
Example endpoint response
/actuator/health
before Spring Boot 2.2 (Actuator V2):
{ "status": "UP", "details": { "db": { "status": "UP", "details": { "database": "HSQL Database Engine", "result": 1, "validationQuery": "SELECT COUNT(*) FROM INFORMATION_SCHEMA.SYSTEM_USERS" } }, "diskSpace": { "status": "UP", "details": { "total": 250685575168, "free": 32597131264, "threshold": 10485760 } }, "ping": { "status": "UP" } } }
Endpoint answer
/actuator/health
Spring Boot 2.2 (Actuator V3):
{ "status": "UP", "components": { "db": { "status": "UP", "details": { "database": "HSQL Database Engine", "result": 1, "validationQuery": "SELECT COUNT(*) FROM INFORMATION_SCHEMA.SYSTEM_USERS" } }, "diskSpace": { "status": "UP", "details": { "total": 250685575168, "free": 32605003776, "threshold": 10485760 } }, "ping": { "status": "UP" } } }
Your toolkit may depend on the health actuator V2 format.
Spring Boot 2.2 health is backward compatible with specifying the
Accept
HTTP header: with V2 media type,
application/vnd.spring-boot.actuator.v2+json
You can do it yourself using curl:
curl -H "Accept: application/vnd.spring-boot.actuator.v2+json" http://localhost:8080/actuator/health
Along with this change, it is now also possible to organize
performance indicators into groups .
RSocket Support
RSocket is a binary communication protocol used to transport byte streams. It enables symmetric interaction models through asynchronous messaging over a single channel.
Along with a new starter for RSocket, an extensive auto-configuration was added.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-rsocket</artifactId> </dependency>
For more information, read the
RSocket Spring Boot documentation.
Java 13 support
Java 13 was released on September 17, 2019.
Spring Framework 5.2 and Spring Boot 2.2 now support Java 13.
Java LTS versions 8 and 11 will remain compatible with Spring Boot 2.2.
Kubernetes Cloud Platform Discovery
ConditionalOnCloudPlatform
now determines whether your Spring Boot application is running in Kubernetes.
package nl.jtim.spring.boot; import org.springframework.boot.autoconfigure.condition.ConditionalOnCloudPlatform; import org.springframework.boot.cloud.CloudPlatform; import org.springframework.stereotype.Service; @Service @ConditionalOnCloudPlatform(CloudPlatform.KUBERNETES) public class MyVeryCoolService { }
Banners
Spring Boot comes with a default banner, which is displayed in the console immediately after the application starts.
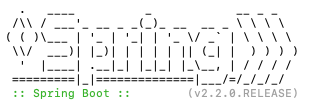
This is a pretty geeky feature, but Spring Boot already supports (animated) custom banners.
Starting with Spring Boot 2.2, you can make your banner even nicer:
- ASCII banner files can now use ANSI 256 color control characters using {AnsiColor.NNN} (where NNN is the color code ).
- Now for (animated) graphic banners, you can set the
spring.banner.image.bitdepth
property to 8
. And the spring.banner.image.pixelmode
property in block
for using block ASCII characters.
The result looks something like this:
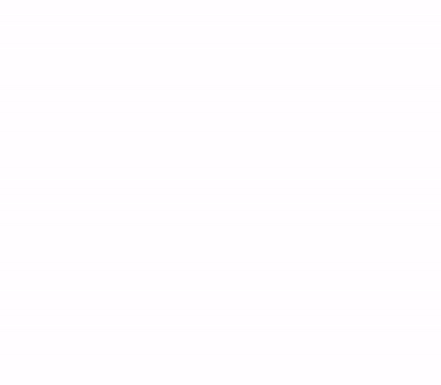
Source:
https://github.com/spring-projects/spring-boot/wiki/images/animated-ascii-art-256.gifFor a simple example of an improved animated Spring Boot 2.2 banner, see
my Github example .
Migrating from Java EE to Jakarta EE
Where possible, the Spring (Boot) command switched from Java EE dependencies with a group identifier of
javax
. Jakarta EE equivalent dependencies with group ID
jakarta
. in Spring Boot starters.
In addition, dependency management for the Jakarta EE dependency API was added along with the existing dependency management for the Java EE dependency API.
Keep in mind that Java EE API dependency management will be removed in future versions of Spring Boot, and it is recommended that you start using the Jakarta EE API dependencies.
Configuration Key Changes
Spring Boot 2.2 introduces many new configuration keys. There are also keys that are outdated and deleted. There are too many changes to cover all of them, but here are some important ones:
logging.file
been renamed to
logging.file.name
logging.path
been renamed to
logging.file.path
For a complete overview of all changes, see
the Spring Boot 2.2 configuration change log!Outdated
Check out the release notes for a complete list of
deprecated classes and properties .
Some changes that should be noted:
- Joda time support deprecated in favor of
java.time
- Elasticsearch transport client and Jest client are deprecated in favor of other options such as RestHighLevelClient and ReactiveElasticsearchClient, see the documentation for more details.
Dependency Updates
Spring Boot 2.2 comes with many updated dependencies.
Spring Dependency Updates:The most important updates to other dependencies:Test dependency update:Upgrading to Spring Boot 2.2
Since August 1, 2019, Spring Boot 1.x has
completed its life cycle . If you are still using Spring Boot 1.x applications, it's time to upgrade!
Remember that classes, methods, and properties that are deprecated in Spring Boot 2.1 have been removed in Spring Boot 2.2. Make sure you don't call deprecated methods before upgrading. Check out
the release notes for deprecated in Spring Boot 2.1.
You can find more information at the following links:
Click + if you found this article helpful!
Have questions or feedback?
Search Twitter:
@TimvanBaarsen