Compiling programs with a text editor using Notepad ++ as an example

Objective: Learn to compile programs from the command line. Learn how to compile from a text editor Notepad ++ (or rather learn how to write scripts and link them), create scripts and macros, set system variables.
The text editor itself does not compile or run anything. Also, the IDE does not compile. Compilation is done using the compiler. Execution is done by some runtime. It can be a command interpreter or runtime.
When compiling, C # programs are packaged in assemblies. IL code is automatically converted to code for a specific processor. As for java, the compiled .class files are executed by the Java virtual machine. .Java files are compiled into bytecode, i.e. some intermediate code. Compilation in an exe file is also performed by the compiler.
.EXE (abbr. English executable - executable) - extension of executable files used in operating systems DOS, Windows, Symbian OS, OS / 2 and in some others, corresponding to a number of formats. The process of obtaining an exe file consists of the following steps: preprocessing, assembly, compilation, linking.
OUT files - executable files for UNIX-like operating systems.
A file can store executable code, a shared dynamic library, or object code.
Terminology
Cmd.exe is a command line interpreter for the OS / 2, Windows CE operating systems and for the family of operating systems based on Windows NT (Windows NT-based). cmd.exe is an analogue of COMMAND.COM
A text processor is a computer program used to write and modify documents, layout text and preview documents in the form in which they will be printed (a property known as WYSIWYG).
Word processors, unlike text editors, have more options for formatting text, embedding graphics, formulas, tables and other objects in it.
A script (script) is a separate sequence of actions created to automatically complete a task.
The user interface , also known as the user interface (UI) is an interface that provides information transfer between the human user and the hardware and software components of a computer system (ISO / IEC / IEEE 24765-2010).
GUI stands for graphical user interface.
A GUI consists of one or more windows and each window contains one or more controls.
SDK (from the English software development kit) is a set of development tools that allows software specialists to create applications for a specific software package, basic development software, hardware platform, computer system, game consoles, operating systems and other platforms.
IDE (Integrated Development Environment) is an integrated software development environment.
An environment variable is a text variable of the operating system that stores some information - for example, data about system settings.
Windows environment variables fall into two categories:
User environment variables - indicate the path to user directories.
System variables - store data about some directories of the operating system and computer configuration.
Background
To build projects, the command line is often used now.
How does this simplify assembly? The fact is that you can create a batch file with compiler options, with logging options, with error settings and many other parameters that will automate your build. The advantages of this approach are obvious:
You don’t waste time loading a heavy IDE, you can automate many details of your assembly. From the command line, program arguments that are not available from the GUI can be accessed. A GUI usually has fewer features, because not all commands and arguments will be provided to the user. You can write your script (a set of commands, logic and variables) into a separate file and run if necessary.
Moreover, this approach will give you a deeper study and understanding of what happens at runtime. You can consider each step separately. Another aspect, using notepad for compilation, provides you with the ability to debug and run small pieces of code without using your heavy IDE. You may not have the IDE installed at the right time and may not have an Internet connection. Basically, you can use another text editor, not Notepad ++. The basic idea remains the same. In this article I will describe the use of cmd Windows 10, Notepad ++ and the NppExec plugin. In previous versions of Windows, this also works. There may be slight differences. You can figure this out on your own.
Note that this is not a call to abandon convenient IDE tools. Good, convenient IDEs increase the productivity of the development team. Unfortunately, for one reason or another, it is not always possible to use them.
You can download programs from official sites:
Notepad ++:
notepad-plus-plus.orgProgram release sources:
github.com/notepad-plus-plus/notepad-plus-plus/releasesProgram author: Don HO, Senior Software Engineer
Biography \ Summary of the author:
donho.imtqy.comPlugin author: Vitaliy Dovgan
Help: Don HO, Jens Lorenz, Juha Nieminen, Joel Yulilouma
You can
download the new version of NppExec
from the site .
Newer versions of Notepad ++ support installing plugins from repositories. You just need to click on the plugins / plugin management menu, type NppExec in the search bar, check the box and click install. If you are using an earlier version of npp, then you should download the plugin and unzip it into the plugins folder. Usually it is on the way ... Notepad ++ \ plugins.
NppExec Versions: Unicode and ANSI
The developer recommends using the Unicode version.
Find out the version of NppExec: Plugins \ NppExec \ Help / About
First, consider compilation from the command line.
The cmd program on Windows is located in the following path:
%windir%\system32\cmd.exe
You can run the program by clicking on the cmd search and clicking on the “command line” application or from the “run” menu by typing cmd.
Cmd console settings

In the upper left corner, click on the console icon and select “Properties” to change the current properties. You can change a number of console properties: color, font, layout.
To change the default properties, select "default values."
You can assign hotkeys to invoke the command line.
To do this, type cmd in the search, right-click on "Command Prompt" and select "go to file location. Then, right-click on the location and properties. Place the cursor in the "Quick Call" field and press the key combination on the keyboard, for example: Ctrl + Alt + T. This keyboard shortcut corresponds to a Linux Ubuntu terminal call and you won’t need to learn anything new. If you want, you can set your own keyboard shortcut. cmd will be called on behalf of the user. To run cmd as administrator, type cmd in the search, right-click on it and select "Run as administrator".
cmd.exe - file, this is part of the system, the shell of the Windows system.
Command interpreter, command line interpreter - a computer program, part of the operating system that provides basic computer control capabilities through interactive input of commands through the command line interface or sequential execution of batch batch files.
Startup keys cmd.exe:
/ c executes the command specified by the line and terminates the application;
/ k executes the command specified by the line and continues the application;
/ a - sets the output to the ANSI standard;
/ u - sets the output in Unicode;
/? - output help on the command line;
To compile from the command line, you need to know a minimal set of commands.
For a deeper study, you will most likely need concepts such as:
Processes, Threads, Streams, Fibers, semaphores, mutexes and so on. All that is connected with multithreading and multitasking.
In this short article, for now, such deep knowledge is not required.
A little bit about variablesYou can use variables that relate to user environment variables, cmd variables, Notepad ++ program variables, NppExec plugin variables.
Aliases - in general, these are aliases of variables. They can be used to specify a short variable name in a specific scope.
In our context, the word “team” means a team or program. You can get a list of commands by typing "help" on the command line without quotes. A list of commands is displayed with their description. You can get help with a specific command by typing help command_name.
Compiling java programs from the command line
To compile java programs, jdk must be installed on your computer.
“The Java Development Kit (JDK for short) is a free Java application development kit from Oracle Corporation (formerly Sun Microsystems) that includes the Java compiler (javac).” The JDK includes standard Java class libraries, examples, documentation, various utilities, and the Java Executive System (JRE). The JDK does not include an integrated Java development environment, so a developer using only the JDK is forced to use an external text editor and compile their programs using command-line utilities. You can download it from the Oracle website, or select OpenJDK. There are third-party Java application development kits available for a number of platforms. Link to download jdk:
jdk.java.net/archiveHere are a few jdk currently in existence: Oracle JDK, OpenJDK by Oracle, AdoptOpenJDK, Red Hat OpenJDK, Azul Zulu, Amazon Corretto. Download and install, everything is described in detail in the installation documentation. If you are already using IDE - an integrated development environment with the JDK already installed, then you just have to find it on your disk. Development environments either include one of the JDK versions in the package, or require JDK to be installed on the developer's machine for their work. For compilation and execution, you need the files: javac.exe - for compilation and java.exe - for program execution. Note jdk must be installed because a number of resource files are used during compilation and execution, such as class libraries, debugger, and other resources.
Compilation from the command line in a simplified form looks like this:
javac HelloWorld.java
. Why simplified? Because we do not specify the full path to the compiler, the full path to the file, do not specify other parameters, such as the name of the output file and others.
If you simply execute such a command without first configuring anything, then most likely you will get an error: "javac is not an internal or external command, an executable program or a batch file." That is, your system "does not know" where javac is and we need to explicitly indicate where the compiler is located.
Read about installing java
in the official documentation .
Where java is installed, you can find out by typing the command in the console:
where java
We are writing a new line:
c:\___javac\javac HelloWorld.java :\___\___\...\javac HelloWorld.java
Here folder_on_ drive_first, folder_on_ drive_second is the name of your folders on disk.
That is, this is the full path to the javac file.
Most likely you will get the following error:
javac: file not found: hello.java Usage: javac <options> <source files> use -help for a list of possible options
In this case, javac tells us that there is no such hello.java file and offers to get acquainted with the compiler options.
We can solve this problem in two ways. Go to the directory in the terminal.
cd ____java_
Or specify the full path when compiling.
:\___\___\...\javac :\\HelloWorld.java
A clarification should be made here, in this case I am considering the path on Windows systems.
In other systems, paths are written differently. See the documentation for more details.
In case of successful compilation, the HelloWorld.class file will appear in your directory
We can execute this file with the command:
:\___\___\...\java -classpath . HelloWorld
In this case, the dot means the current package, the current directory.
If -classpath is not specified, error output is possible:
«Error: Could not find or load main class »
If you specify a package in the class, then when you run the compiled file you need to specify the path to the package.
We figured out a bit how to compile and run java programs from the command line. Now we need to do the same in Notepad ++ using the NppExec plugin. Download and install the plugin, if it is not already installed and install.
After proper installation, the NppExec plugin will appear in the Plugins menu.
NppExec has its own console like CMD.
By default, the command prompt is not displayed. To show \ hide the console, select: Plugins \ NppExec \ Show console.
For help, type help in the console and press Enter. For more information about NppExec, type in the console: help npp_exec.
From help:
So, generally speaking, NppExec is a tool. This tool does exactly what you say, without any assumptions, without implicitly invoking anything, and so on.
This can be critical during development when the “native”, “smart” console “guesses” something, for example, in which system it is running and can substitute its “smart” values.
Do _not_ use NPP_EXEC to start a batch file or an executable file in NppExec!
Do not use the npp_exec command to start an exe file or bat file.
The purpose of NPP_EXEC is to execute its own script.
To start a third-party application, type:
application.exe // in this case exe file named application.exe
batchfile.bat // in this case, batch named "batchfile.bat"
We can only create settings for compilation and execution. We will make these settings separately. Create the settings for compilation and create the settings for compilation and execution.
Setting system variables
In order not to enter the full path to the program each time, be it a compiler,
linker, build system, etc., you can set system variables.
How are system variables set? You can set them in the GUI, from cmd, using Power Shell, using regedit.
How to set system variables using the graphical interface
Computer Properties \ Advanced \ Environment Variables \ User Environment Variables for "username". Here you can create or edit an existing environment variable.
How to set an environment variable using the command line
To set environment variables, you need to have administrator rights.
md
SET
- output of the current installed variables of the system environment. The command does not display a complete list.
Other variables take their values dynamically:
% CD% - takes the value of the current directory.
% DATE% - takes the value of the current date.
% TIME% - takes the value of the current time.
% RANDOM% is a random number between 0 and 32767.
% ERRORLEVEL% - the current value of ERRORLEVEL, a special variable that is used as a sign of the result of program execution.
% CMDEXTVERSION% value of the extended command processing version of CMD.EXE.
% CMDCMDLINE% -
Expands to the original command line that invoked the command processor.
SET =
variable - The name of the environment variable.
string - A string of characters assigned to the specified variable.
set _
- Shows the current value of the variable named variable_name.
echo %_%
- Displays the value of a variable.
The variables specified by the set command are valid only for the duration of the command session in which they were set. That is, the variables will be valid until the current user session ends or until the reboot.
In order for the variables to work after a reboot, you need to add them to the system registry (In the case of using the Windows line of systems). They can be added using the Windows GUI:
Choose Run or Run (Win + R key)
control sysdm.cpl
You will see the system properties window. Select Advanced and Environment Variables.
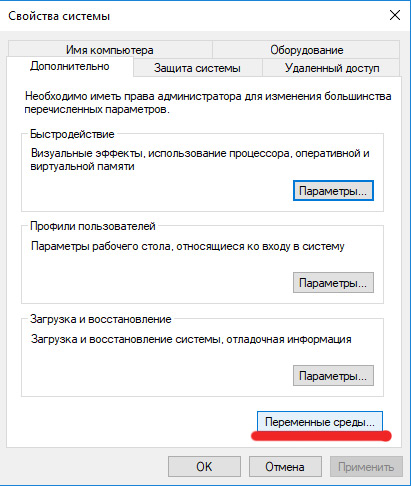
There are two kinds of environment variables here. These are system variables and user environment variables. User environment variables refer to a specific user of the system and its system environment. Here you can set the Path variable for quick access to frequently used programs. The value of the variable is a string.
This is a set of paths separated by a ";"
An environment variable is a text variable of the operating system that stores some information - for example, data about system settings. Environment variables are set by the user or shell scripts.
You can also do this using the command line utility for working with the Windows registry -
reg .
reg /?
- getting help on this utility.
REG ADD <_> [/v <_> | /ve] [/t <>] [/s <>] [/d <>] [/f] [/reg:32 | /reg:64]
An example of using a script to add a system variable:
SET KEY=____ SET PARM=_
These variables are saved until the end of the current command session or until the value changes in the current session.
To add the path variable for the current user (the current user is the user whose name you used when entering the session):
We set the values of the variables and use them in the command
REG ADD ...
The Path value indicates the path through the semicolon.
SET KEY=HKEY_CURRENT_USER\Environment SET PARAM=%systemdrive%\;d:\; REG ADD %KEY% /v Path /d %PARAM%
We need to indicate in the PARAM value all the paths that we have already installed and the path to the compiler.
HKEY_CURRENT_USER is the current user branch in the registry.
Environment - environment variables are stored here.
Warning: “This is how you work with the registry. Be sure to make a copy of the registry and create a system restore point for your system. ” It will be safer to set system variables and user environment variables using regular means.Be sure to check out the materials:
How to back up and restore the registry in WindowsApplies to: Windows 7, Windows 8.1, Windows 10 (Official Microsoft Support):
support.microsoft.com/en-us/help/322756Creating a system restore pointApplies to: Windows 10
support.microsoft.com/ru-ru/help/4027538/windows-create-a-system-restore-point mrt
- launch the Malicious Software Removal Tool
NppExec Settings in Notepad ++
Open Nonepad ++.
We need to create cmd scripts.
Select Plugins \ NppExec \
Disable "Console Command History";
Turn on “Save all files on execute”;
Turn on “Follow $ (CURRENT_DIRECTORY)”
Select Plugins \ NppExec \ Execute or press F6.
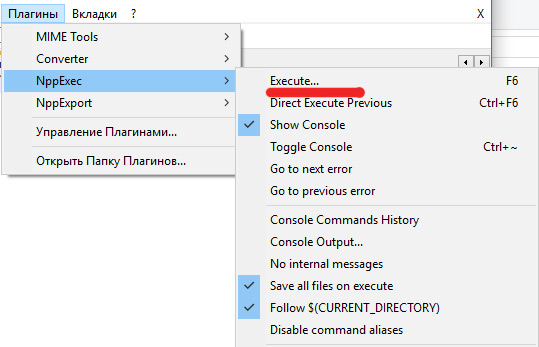
In the window that opens, write:
cd "$(CURRENT_DIRECTORY)" javac $(FILE_NAME)
Click Save ... and in the field ScriptNme write: CompileJava.
This script will execute our java code.
Again select Plugins \ NppExec \ Execete or press F6.
cd "$(CURRENT_DIRECTORY)" java $(NAME_PART)
Click Save ... and write the name "RunJava". This code, written above, will compile Java code.
We repeat the same thing and write (For compilation and execution):
cd "$(CURRENT_DIRECTORY)" javac $(FILE_NAME) if $(EXITCODE) !=0 goto exit java $(NAME_PART) :exit
Save as CompileAndRunJava.
Click the OK button.
cd "$(CURRENT_DIRECTORY)"
- go to the current folder. In this case, the folder where you saved the text file will be current.
javac $(FILE_NAME)
- compile the file with the name located in the FILE_NAME variable.
In our case, this is the name of the saved file. The following is a condition.
if $(EXITCODE) !=0 goto exit
- if our code did not return normal completion == 0, then exit.
java $(NAME_PART)
- execute our compiled file.
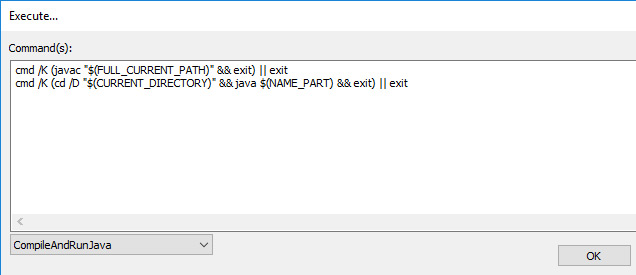
Next we will connect our scripts with macros.
In Notepad ++, select Plugins \ NppExec \ Advanced options ...
Check the box next to “Place to the Macros submenu”. In the “Associated script:” drop-down list, select the script we need and give it a name in the “Item Name” field. And click Add / Modify. We do this for all our scripts, click “OK” and restart Notepad.
Solving problems with incorrect output of Russian letters in the console:
Plugins / NppExec / Console Output
We put the input and output encodings we need.
Disabling internal console messages: Plugins / NppExec / No internal messages.
If a message appears when executing the file OurClassName.class that main was not found, just change our script: That is, we need to specify either the full path to the package or indicate the current package with the symbol “.”.
We get the following script:
cd "$(CURRENT_DIRECTORY)" java . $(NAME_PART)
If cmd does not find the java file, then indicate the full path to it.
DriveName: \ folder1 \ folder2 \ ... \ java. $ (NAME_PART).
We will slightly improve our code:
npp_save cd "$(CURRENT_DIRECTORY)" __javac\javac $(FILE_NAME) if $(EXITCODE) !=0 goto exit __java\java . $(NAME_PART) :exit
Perl compilation script:
npp_save CD $(CURRENT_DIRECTORY) perl.exe -c -w "$(FILE_NAME)"
Script explanation:
npp_save - internal npp command, save
CD $ (CURRENT_DIRECTORY) - go to the current folder
perl.exe -c -w "$ (FILE_NAME)" executing perl.exe -c -w
example: perl.exe -c -w test.pl
-c means compile, compile
-w means warnings; issue warnings
Script for compiling c #:
cd "$(CURRENT_DIRECTORY)" __csc\csc $(FILE_NAME) if $(EXITCODE) !=0 goto exit $(NAME_PART) :exit
Here cc_path is the path to your csc.exe
Usually it looks like this:% systemdrive% \ Windows \ Microsoft.NET \ Framework64 \ version \
For the 32-bit version - Framework32. You can not specify the full path, but add the path to your system variable.
There is such a feature of cmd, when you change the path and specify a different drive, then you can see the same path. Nothing seems to happen when executing the command:
CD _:\1\2
.
To change the disk, you need to use a CD with the / D switch or explicitly specify the drive_name: after entering the command.
Example 1:
cd e:\1\2 e:
Example 2:
cd /D e:\1\2
here e is the drive name that is different from the current one.
Compilation Error Detection Technique
At the stage of preparation, you may experience various kinds of errors, and the first thing you need to find out is what your error relates to. That is, to localize the problem. To do this, we find out at what level the problem arises. Whether it refers to the system, to the command shell, to the compiler, to a text editor or to a typo, an error in the script.
- Check if cmd is running and whether the simplest commands work out.
- Check your compiler for compilation from the command line.
- Check system variables
- Check if NppExec plugin is installed
- Does he run scripts
- Are scripts related to NppExec macros?
- Do macros work together with scripts.
For a more detailed output of compilation errors, see the documentation for your build system, for the compiler, and other modules that you use.
Now that we have created, debugged and our scripts, made sure that they work predictably, created macros for them, we can associate them with shortcuts. How to do it?
We did not assign hotkeys when creating scripts, because their assignment, shortcut keys, in the script creation window is limited.
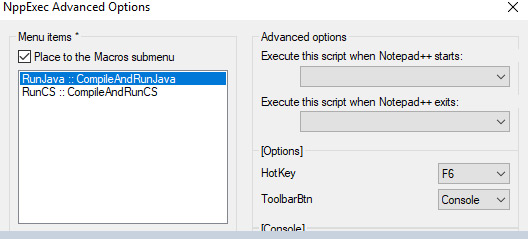
We are not given a large selection of keyboard shortcuts. In addition, these key combinations may be busy with us. To assign hotkeys or shortcut keys, we can in the menu:
Maxros / Change Keyword / Delete Macro .


What reasons might you have for getting to know the command line?You may not have to use it often. But to know about its existence is simply necessary. For example, at the initial stage of training, you need to get acquainted with the options of the compiler, linker, build system and test them. You will need to know what arguments and keys the program accepts at the input and what it can return.
You will not always have the opportunity to use smart and expensive IDEs. And not because they may be too expensive, but for the reason that you simply can not install them on some systems. In other systems, security measures may require this. You may need to test each module, such as a compiler, linker, linker, build system, version control system separately. That is, every part of your development system. You may need to check the correctness of their work separately. In some cases, you may need to use another compiler with your IDE or any other separate module. Collecting GUI cubes in the IDE does not make you a programmer.
You will need knowledge of languages, knowledge of architecture, knowledge of some scripts, mathematical knowledge, etc., that is, the ability to use many tools in the work. In different terminals and consoles, the command line works differently. It depends on your console or terminal. The general principles of work for some are similar, but for some they can differ very much. The command line is only an additional tool in your hands, and how you use it effectively is up to you. In the case when you are engaged in an opensource project, you can provide batch files to your users for assembly, for testing the project, deploying the project, etc. For the development team, this approach can help to interact more effectively. Moreover, this may turn out to be beyond the limits of “comfort” and carry additional risks.But the use of any tools carries risks, in many respects it is determined by the reliability of the tools and the ability to use them.What is the advantage of this compilation approach? For students, this is the acquisition of new command line skills and linking script code with a text editor, learning compilation from the command line. Save time by testing small code without loading heavy IDEs. In some cases, when it is not possible to establish a full-fledged IDE, this approach can help. Studying scripts for the command line allows you to compile from source where there is no possibility at all to use UI, for example, on servers. And although there will not be the possibility to use text editors like Notepad ++, there will be the opportunity to use word processors like vi, vim, emacs, nano, etc. The basic principles of work remain the same.You and I, somewhat briefly, got acquainted with compilation from the command line, with writing scripts for this, with setting and using system variables,with binding script code with Notepad ++ macros, with Notepad ++ internal variables. I hope this article will be useful to students. Here I described in detail the general methods. Before contacting support or sending bugs, you should find out on your own. In many cases, it may turn out to be your mistake or typo, not the development engineers.Modern IDEs have their own consoles or console emulators. So you do not have to use cmd to build. In addition to cmd, there are quite a lot of similar tools. Studying the work of scripts can help you in the future in mastering such tools as build systems, compilers, linkers, linkers. In addition, some SDKs do not provide a graphical user interest (GUI), the use of a GUI may be prohibited by a security policy, as it carries additional threats in critical applications.I hope this publication will be useful in the development, expansion of new knowledge and skills.<<< My publications on Habr >>>useful linksHow to back up and restore the registry in WindowsApplies to: Windows 7Windows 8.1Windows 10 (Microsoft Official Support)support.microsoft.com/en-us/help/322756Materials for further study
notepad-plus-plus.orgManaging the Java classpath (UNIX and Mac OS X)Managing the Java classpath (Windows)Java - the Java application launcherFor reference: Getting ridof the “historical reasons” in cmd.exehabr.com/en/post/ 260991Comparison of shellsru.wikipedia.org/wiki/%D0%A1%D1%80%D0%B0%D0%B2%D0%BD%D0%B5%D0%BD%D0%B8%D0%B5_%D0 % BA% D0% BE% D0% BC% D0% B0% D0% BD% D0% B4% D0% BD% D1% 8B% D1% 85_% D0% BE% D0% B1% D0% BE% D0% BB % D0% BE% D1% 87% D0% B5% D0% BA