はじめに
Active Recordは、データベースを使用して情報を保存するアプリケーションの設計パターンです。 データベーステーブルはアプリケーションクラスとして表示され、クラスオブジェクトの表示はテーブル行です。
このようなオブジェクトのインターフェイスには、Insert、Update、Deleteなどの機能に加えて、テーブルの列に対応するプロパティを含める必要があります。 オブジェクトを作成すると、新しい行がテーブルに追加され、オブジェクトが更新されると、テーブル内の対応する行も更新されます。
コアデータとアクティブレコード
Core DataはActive Recordパターンに基づいていますが、データベースを操作するにはかなり面倒なコードを書く必要があります。 たとえば
、Appleの例を次に示します。
NSManagedObjectContext *moc = [self managedObjectContext]; NSEntityDescription *entityDescription = [NSEntityDescription entityForName:@"Employee" inManagedObjectContext:moc]; NSFetchRequest *request = [[[NSFetchRequest alloc] init] autorelease]; [request setEntity:entityDescription];
例からわかるように、姓に「Worsley」と最低給与を超える給与が含まれるEmployeeデータベースを検索するには、多くの「余分な」コードを記述する必要があります。
Magical Pandaは、
Core Dataライブラリの
ActiveRecordでこの問題の解決策を提供しました。
以下は同じ例で、コアデータにActiveRecordのみを使用しています。
NSNumber *minimumSalary = ...; NSPredicate *predicate = [NSPredicate predicateWithFormat:@"(lastName LIKE[c] 'Worsley') AND (salary > %@)", minimumSalary]; NSArray *employees = [Employee findAllSortedBy:@"firstName" asceding:YES withPredicate:predicate];
コアデータのActiveRecordを使用すると、次のことができます。
1.コアデータを操作するためのクリーンなコードを作成する
2.簡単な単一行のクエリを作成できます
3.シンプルであるにもかかわらず、リクエストを変更する必要がある場合にNSFetchRequestを変更できます。
コアデータにActiveRecordを使用する
カスタマイズ
コアデータライブラリのActiveRecordをダウンロードし、プロジェクトに追加します。
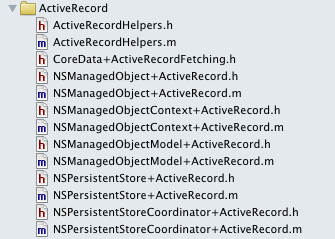
MyProjectName-Prefix.pchに追加
#import "CoreData+ActiveRecordFetching.h"
アプリケーションのAppDelegateで:didFinishLaunchingWithOptions:呼び出しを追加します
[ActiveRecordHelpers setupCoreDataStackWithAutoMigratingSqliteStoreNamed:@"MyProject.sqlite"];
このメソッドは、指定された名前でNSPersistentStoreを作成し、アプリケーションバンドルで検出した.xcdatamodeldファイルをモデル化します。
デフォルトの管理対象オブジェクトコンテキスト
Core Dataを使用する場合、NSManagedObjectContextオブジェクトが使用されます。 コアデータのActiveRecordを使用すると、DefaultContextを設定できます。これは、アプリケーション全体に対してデフォルトでNSManagedObjectContextになります。 他のスレッドで使用するNSManagedObjectContextの作成は次のとおりです。
NSManagedObjectContext *myNewContext = [NSManagedObjectContext newContext];
このコンテキストはデフォルトで作成でき、リクエストメソッドの名前が「inContext:」で終わらない場合、すべてのリクエストで使用されます。 メインスレッドでのみデフォルトコンテキストを作成および設定することをお勧めします。
データサンプリング
id、length、nameの各フィールドを持つクラスSongがあるとします:
@interface Song : NSManagedObject
@property (nonatomic, retain) NSNumber * length;
@property (nonatomic, retain) NSString * name;
@property (nonatomic, retain) NSNumber * id;
@end
#import "Song.h"
@implementation Song
@dynamic length;
@dynamic name;
@dynamic id;
@end
コアデータライブラリのActiveRecordには、カテゴリ「NSManagedObject + ActiveRecord.h」が含まれています。つまり、NSManagedObjectのすべての子孫は、コアデータメッセージのActiveRecordに応答します。
Active Record for Core Dataのほとんどのメソッドは、結果の配列を返します。 たとえば、データベース内のすべてのSongオブジェクトを見つける必要がある場合、呼び出しは次のようになります。
NSArray *songs = [Song findAll];
名前でソートされた曲の検索は次のようになります。
NSArray *songsSorted = [Song findAllSortedByProperty:@"name" ascending:YES];
一意の属性値を持つ曲がある場合、次の機能を使用できます。
Song *song = [Song findFirstByAttribute:@"name" withValue:@"Imagine"];
コアデータのActiveRecordでは、NSPredicateを使用することもできます。
NSPredicate * songFilter = [NSPredicate predicateWithFormat:@ "length>%@"、...];
NSArray *songs = [Song findAllWithPredicate:peopleFilter];
データ編集
ActiveRecord for Core Dataを使用したデータベースレコードの作成は非常に簡単です。
Song *song = [Song createEntity];
取り外し:
[song deleteEntity];
属性の編集:
Song *song = [Song createEntity];
song.name = "stairway to heaven";
song.length = [NSNumber numberWithInt:150];
データを編集したら、コンテキストを保存する必要があります。
[[NSManagedObjectContext defaultContext] save];